There you are. You are studying for a coding interview or trying to stop faking to be a developer while asking silly questions such as how to revert an array to ChatGPT. What do 90% of people do? They go to LeetCode and start planning to complete 100 problems a day. Sound good. Yes, except after the first excitement, you discover that the algorithm you crafted throws an error. Or it exceeds time limits. Or spit out inexplicable outputs. What. The. F.
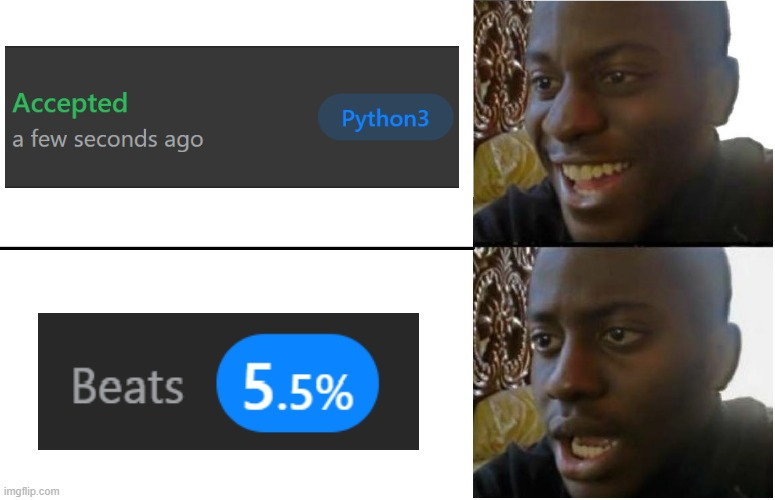
What is Leetcode
For those who do not know it, LeetCode is a popular platform that provides various coding challenges and solutions to help coding enthusiasts, students, and professionals prepare for technical interviews.
This website has a vast community of programmers who come together to solve coding problems, answer each other’s questions, and share their knowledge. LeetCode offers various coding questions frequently asked in technical interviews of top tech companies, including Amazon, Apple, Google, and Microsoft.
LeetCode can be a valuable tool for preparing for technical interviews as it provides a platform for practising coding challenges commonly asked in interviews. By solving these challenges, users can improve their problem-solving skills and gain confidence to tackle similar challenges during interviews.
For example, a familiar coding challenge users might encounter on LeetCode is implementing a function that checks whether a given string is a palindrome. By practising this challenge on LeetCode, users can become more familiar with standard programming techniques and better understand how to approach similar problems.
If you already understood that copy-pasting someone else’s solution does count, you should debug your code. And in this moment of desperation, LeetCode throws you the paywall. 29$ dollar/month, and you’ll unlock incredible powers to debug your code, finally find what was not working as expected, and revert that fricking array. Shall we start?
Pre-requisites
The following is my proposal or prerequisites, but there are many other ways. You can find guides on installation in the links below:
How to
Let’s say you are working on the two-sum problem, which asks us to return indices of the two numbers such that they add up to target
Given an array of integers nums
and an integer target.
You can start copy-pasting the code into VS Code with your solution (yeah, I did not want to spoil it there!)
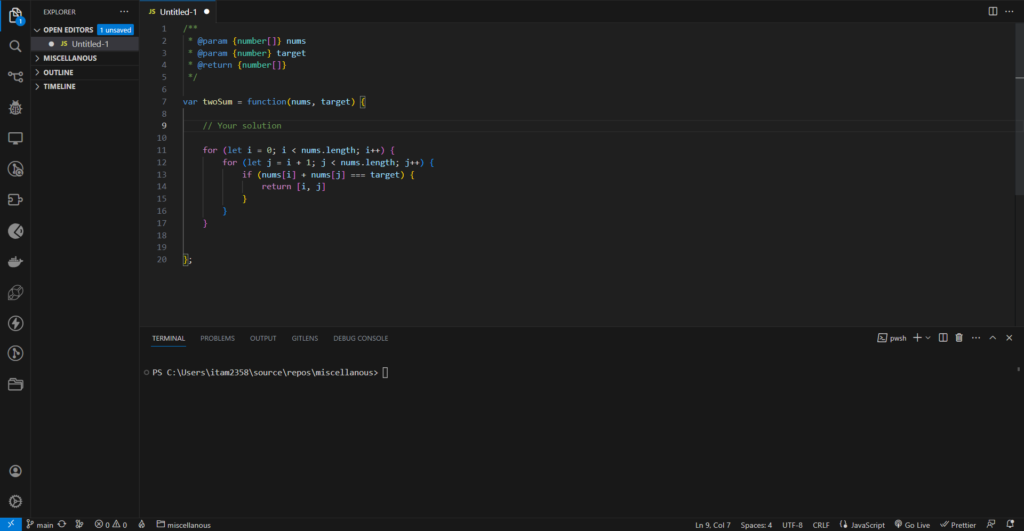
After that, add the test case in your code with a simple console log (or Console.WriteLine() or print(), according to your chosen language). For instance, for the following case, let’s log twoSums([2,7,11,15], 9)
.
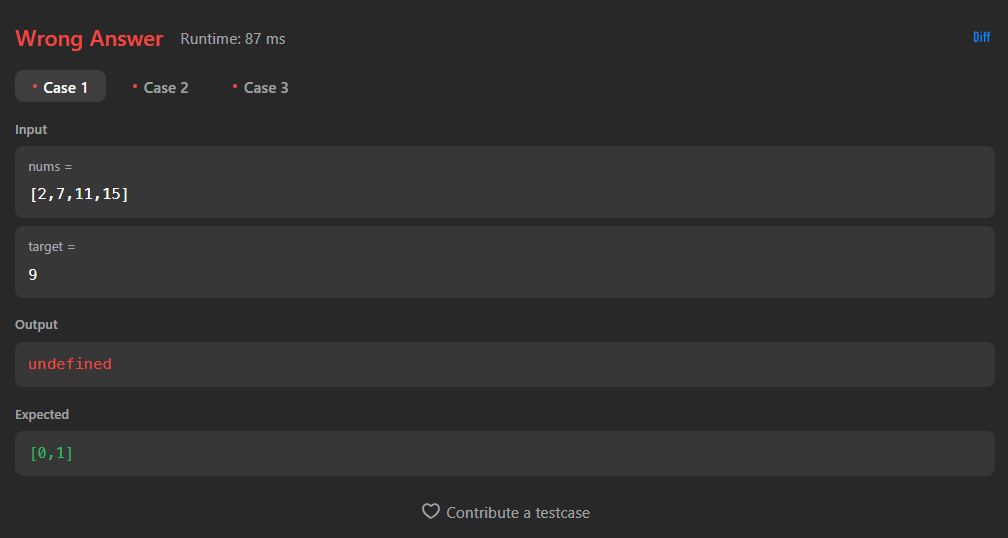
After that, start debugging, adding a breakpoint on the left bar and click “Run and Debug” with Node, as in below.
Using a debugger when checking algorithms in JavaScript is helpful because it allows you to step through the code line by line and see what’s happening at each step. This can be especially helpful when dealing with complex data structures or when trying to pinpoint specific errors in your code. Some of your powers when using a debugger instead of console logging everything include setting breakpoints, which allow you to pause the code at specific points and examine the values of variables and data structures at that moment. You can also step through the code one line at a time, look at call stacks, and watch expressions, which allow you to see the value of an expression at any point during the execution of the code.
Debuggers can also help you identify and fix issues with asynchronous code, such as race conditions or infinite loops. In summary, debuggers offer robust tools for identifying and resolving issues in your code, making them an essential tool for any developer.
Debug complex data structures
Good, now you can check variable values anytime and figure out where you may have made wrong assumptions.
But what if you are working on a complex data structure, such as a binary tree? You cannot initialise one by saying let tree = new BinaryTree()
because Javascript and many other programming languages do not have a Binary Tree type. But it’s okay for us! We can generate a class and initialise it so you can finally deal with the tedious problem of balance check of a binary tree.
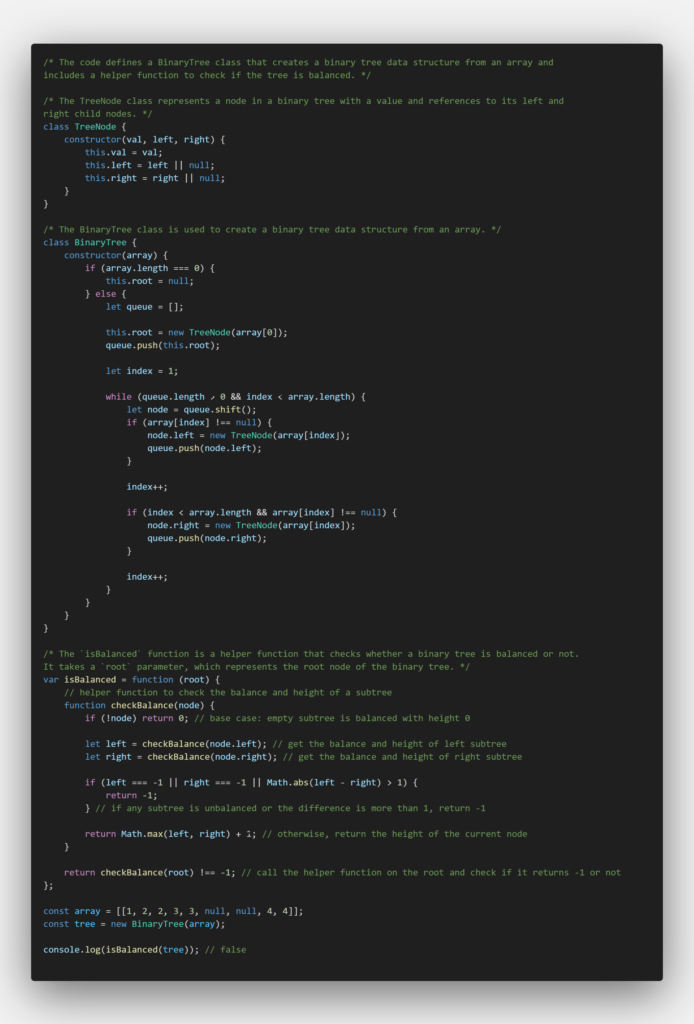
All in all
In conclusion, LeetCode can be a valuable resource for coding enthusiasts, students, and professionals preparing for technical interviews. When encountering errors or unexpected outputs, using a debugger becomes essential. Debuggers like the one provided by Visual Studio Code allow you to step through the code line by line, examine variable values, set breakpoints, and analyze complex data structures.
While LeetCode provides many coding challenges, it’s important to note that some problems may involve complex data structures, like binary trees, that may not have built-in support in certain programming languages. In such cases, you can generate a class or implement your solution to handle these structures effectively.